The app is now finished - check it out here: https://www.monicaspastrycakes.com/
This post is about React hooks, particularly the state hook, and how it was used in my recent project.
React Hooks
What is a hook?
If you write a function and realize you need to add some state to it, you have to convert it to a class. React hooks save the trouble of this implementation change by allowing you to simply "hook into" the existing function. Let's see how this is done with the state hook.
How I used the state hook in my app
My application required customers to select options to personalize their orders. In order to do this, I used a variable called productDetails which had child attributes such as flavor, shape, and description. Attributes of productDetails needed to be set dynamically - every time a customer selects something for their desired product. The example below shows how I accomplished this:
State hook example
First, we import the useState method with:
import React, { useState } from 'react'
Next, we define the variable whose state will be updated. We pass initial values for each attribute to the useState method, as so:
const [state, setState] = useState({initial value})
Example from my project:

Now we can call setState to 'update' the state of our variable, as so:
setState({ ...state, state.attribute: attribute_value })
Code walkthrough from my project:
Flavor function
Each flavor is mapped to a picture of the flavor.
Each picture is displayed in a carousel with a caption of the flavor.
When the picture or caption is clicked or selected, we call the PickFlavor.
PickFlavor function
Set the flavor attribute of the productDetails variable by calling setProductDetails.
If the caption (<p> element) is clicked, pass in the innerHTML.
If the picture is clicked, pass in the alt text of the image.
Lastly, a notification pops up to the user.
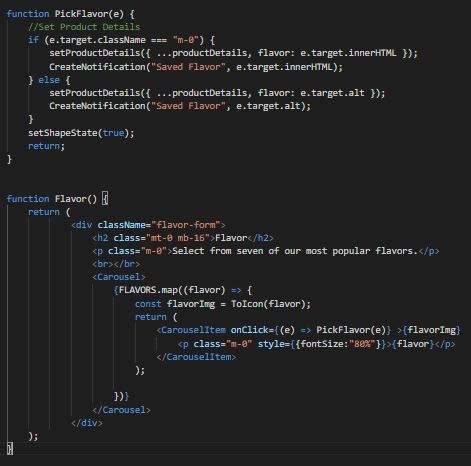
Output:
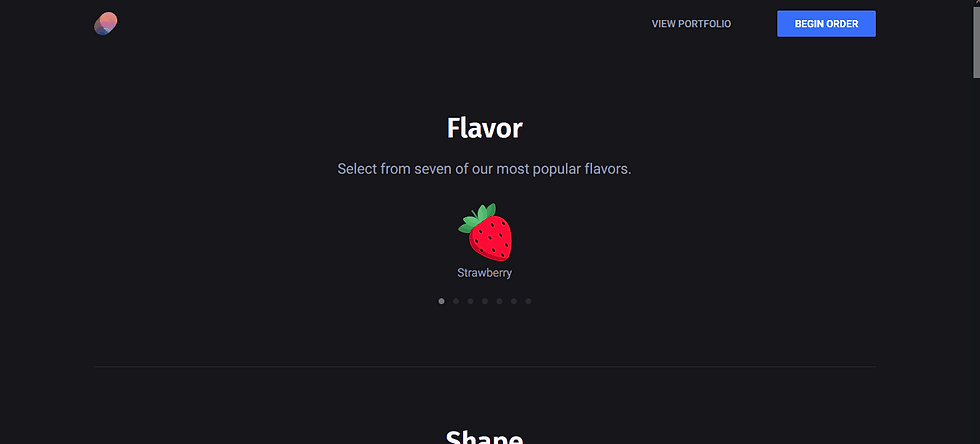
Comentarios